Middleware support for Azure Functions
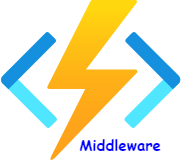
About this blog
Hey folks!, In this blog we will see about a library AzureFunctions.Extensions.Middleware that I developed to make use of middleware pattern to address some of the cross-cutting concerns of our applications.
Middleware support in Azure Functions
Historically we always have known .NET Azure Functions have been in the in-process mode. Until the release of .NET 5, now there are two modes in which we can run Azure Functions
- Out-of-process(Isolated)
- In-process
In the out-of-process mode we have native support for middleware as per the design ( we have direct control over the execution of our function app, which runs as a separate process). But in the In-Process mode there is no middleware capability as we don’t have full control over the application’s startup and dependencies it consumes.
Find more details here
AzureFunctions.Extensions.Middleware
🛑 It is now obvious that we won't be having a direct way to access the pipeline of execution in the traditional In-Process mode which most of us are using in the production environments.
Supported frameworks
AzureFunctions.Extensions.Middleware is supported by below frameworks
- NetCoreapp 3.1
- Net 5.0
- Net 6.0
Install NuGet package
|
|
Add HttpContextAccessor to service collection
Inorder to access/modify HttpContext within custom middleware we need to add HttpContextAccessor in Startup.cs file.
|
|
Add custom middlewares to the pipeline
One or more custom middlewares can be added to the execution pipeline using MiddlewareBuilder.
|
|
Use()
- Use() middleware takes custom middleware as parameter and will be applied to all the endpoints
UseWhen()
- UseWhen() takes Func<HttpContext, bool> and custom middleware as parameters. If the condition is satisfied then middleware will be added to the pipeline of exectuion.
IMiddlewareBuilder dependency
We can now add IMiddlewareBuilder as a dependency to our HTTP trigger function class.
|
|
Execute pipeline
Now we need to bind last middleware for our HttpTrigger method , to do that wrap our existing code inside Functionsmiddleware block “_middlewareBuilder.Execute(new FunctionsMiddleware(async (httpContext) =>{HTTP trigger code})”
|
|
How to contribute?
If you wish to contribute to this open source or provide feedback, Feel free to reach out to me on below links
Github Source code
Leave a ⭐ in the below github repo if this library helped you at handling cross-cutting concerns of the application.
https://github.com/Cloud-Jas/AzureFunctions.Extensions.Middleware
Special thanks
Join us
Please go ahead and join our discord channel (https://discord.gg/8Cs82yNS) to give some valuable feedbacks
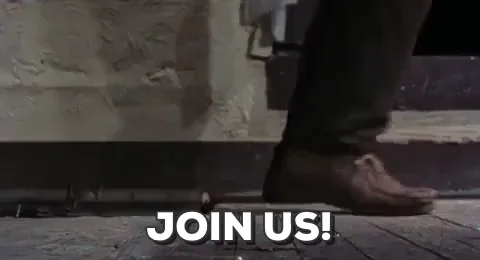